Java is a powerful programming language known for its robustness, portability, and efficiency. Among its many advanced features, concurrency and multithreading play a crucial role in optimizing performance, especially in modern applications. Whether you’re developing a simple application or working on complex enterprise-level projects, understanding how to implement concurrency and manage multithreading in Java is essential. For those looking to gain expertise in this area, structured learning through resources like Java Training in Noida can be invaluable.
Understanding Concurrency in Java
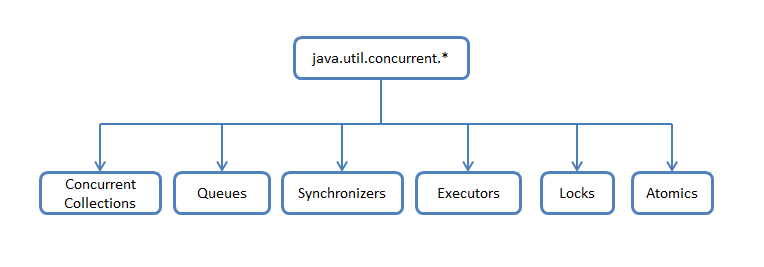
Concurrency in Java refers to the ability to execute multiple sequences of instructions simultaneously. It improves the performance of applications by utilizing CPU resources more efficiently. In concurrent programming, different parts of the program are executed in parallel, resulting in faster processing times, especially for CPU-bound tasks.
Java provides a rich set of APIs for handling concurrency, including java.util.concurrent package, which simplifies writing complex, multithreaded programs. This package includes tools like ExecutorService, Locks, and Futures, making it easier to implement thread management, synchronization, and inter-thread communication.
The core advantage of concurrency is that it enables better resource utilization by breaking down tasks into smaller units that can run independently or parallelly. However, it also introduces challenges such as deadlocks, race conditions, and thread starvation, which require careful handling through synchronized code and thread-safe collections.
As Java continues to dominate in enterprise-level development, expertise in these areas remains a highly sought-after skill. Additionally, learners can also opt for a Java Online Course to practice multithreading at their own pace.
Multithreading in Java

Multithreading is a subset of concurrency that involves running multiple threads within a single process. Java supports multithreading natively through the Thread class and the Runnable interface. Each thread runs independently, but shares the same memory space, making it an efficient method of running multiple tasks simultaneously.
Concepts in Multithreading
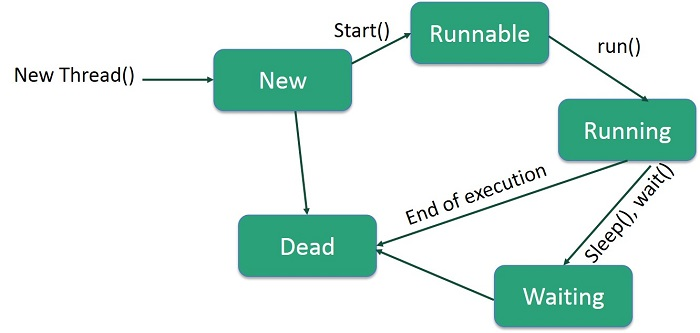
- Thread Life Cycle: A Java thread goes through several states: New, Runnable, Blocked, Waiting, Timed Waiting, and Terminated.
- Creating Threads: Extending the Thread class or implementing the Runnable interface, both methods offer flexibility in defining the task logic within the run() method.
- Thread Synchronization: When multiple threads access shared resources, synchronization is necessary to avoid inconsistent data states. Java provides synchronized blocks and methods to ensure that only one thread can access critical sections of the code at a time.
- Thread Communication: Java provides methods like wait(), notify(), and notifyAll() to facilitate communication between threads, helping manage scenarios where one thread’s action is dependent on another.
Advantages of Multithreading
- Improved Performance: By dividing a task into multiple threads, the CPU can execute parts of the task in parallel, speeding up overall execution.
- Responsiveness: Multithreading allows UI threads to remain responsive while background tasks execute, improving the user experience.
- Better Resource Utilization: Multithreading optimizes resource utilization by allowing the system to work on multiple tasks concurrently.
However, mastering these concepts requires not just theoretical knowledge but hands-on experience, which can be gained through a Java Online Course.
Implementing Multithreading in Java
Example: Creating and Managing Threads
Here’s a simple example of creating and managing threads using the Runnable interface:
class Task implements Runnable {
@Override
public void run() {
System.out.println(Thread.currentThread().getName() + ” is running.”);
}
}
public class Main {
public static void main(String[] args) {
Thread t1 = new Thread(new Task(), “Thread-1”);
Thread t2 = new Thread(new Task(), “Thread-2”);
t1.start();
t2.start();
}
}
In the above example, two threads are created and executed concurrently, each running an instance of the Task class.
Concurrency Challenges and Solutions
Concurrency is powerful but comes with certain challenges, such as:
- Race Conditions: When two or more threads try to access and modify shared data simultaneously, leading to inconsistent results. To avoid this, developers can use synchronized methods or blocks.
- Deadlock: Blocking forever of two or more threads, each waiting on the other. Deadlocks can be avoided by careful design, ensuring that locks are always acquired and released in a consistent order.
- Thread Safety: Data inconsistency can arise when multiple threads read or write shared data concurrently. Using thread-safe data structures like ConcurrentHashMap or ensuring atomicity through the AtomicInteger class can mitigate this issue.
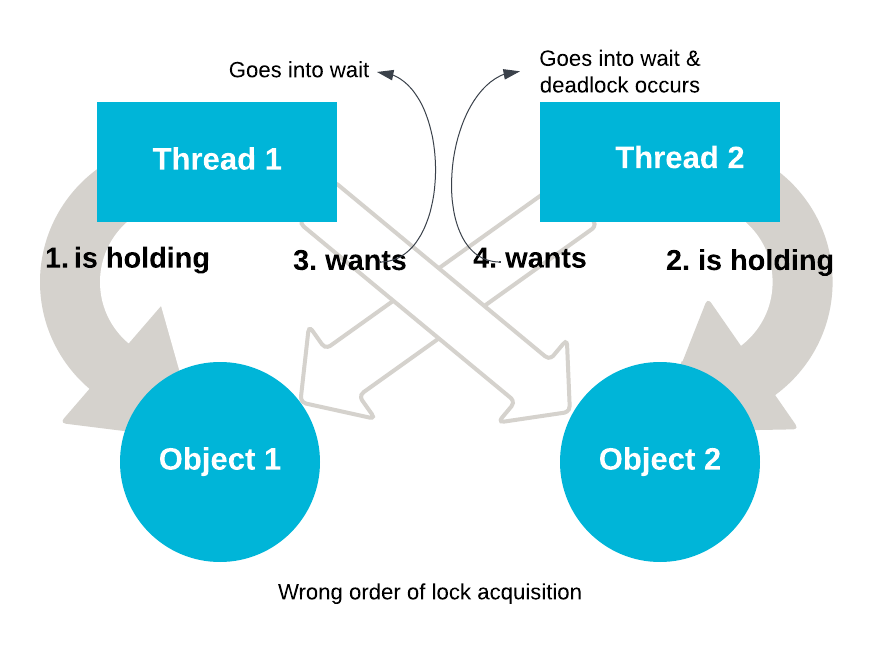
For in-depth understanding and practical knowledge, many learners turn to Java Training in Noida, where experienced instructors teach the nuances of these challenges and provide strategies for solving them.
Java Concurrency Tools
Concurrency Tool | Description | Use Case |
ExecutorService | Manages the lifecycle of threads efficiently | For handling thread pools |
Future | Represents the result of an asynchronous computation | To handle asynchronous task results |
CountDownLatch | Synchronizes multiple threads | To wait for threads to complete tasks |
Semaphore | Limits the number of threads accessing a resource | For managing resource access |
CyclicBarrier | Synchronizes threads at a common barrier point | To wait until all threads reach a point |
Java Concurrency Tool Usage by Industry
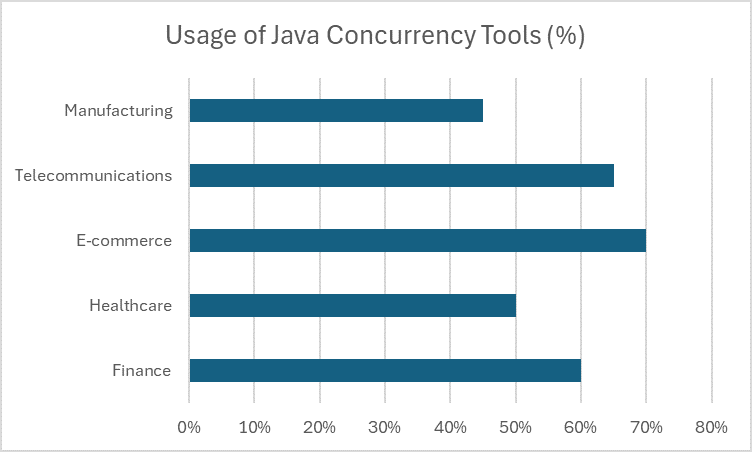
Conclusion
Mastering Java concurrency and multithreading is crucial for building efficient, responsive, and scalable applications. Understanding advanced concepts such as thread synchronization, communication, and managing concurrency tools can significantly enhance your software’s performance. Structured learning through Java Training or a Java Course can provide in-depth knowledge and practical experience in implementing these concepts, preparing you for real-world applications.